Frontend Integration Collection: SvelteKit | GoodData
[ad_1]
Integrating GoodData into SvelteKit is simpler than it appears, even with out native help. Let’s dive in and go over the 4 step course of:
- Putting in Dependencies
- Organising Surroundings Variables
- Organising the Code Base
- Managing CORS
Be aware: For these involved in a deeper dive into these integrations, I like to recommend testing Patrik Braborec’s fast overview.
On the finish of this tutorial, it is possible for you to to combine GoodData Visualizations into your SvelteKit software. For the needs of this demo, let’s assume that you’ve a fruit retailer like this:

That is a part of an ongoing sequence about completely different front-end frameworks and their integration into GoodData, final one was about Angular.
Step 1: Set up Dependencies
First issues first, you would wish so as to add just a few issues to your dependencies.
npm set up --save react@^18.2.0 react-dom@^18.2.0 @gooddata/sdk-ui-all @gooddata/sdk-backend-tiger
Particulars in regards to the GoodData.UI SDK shouldn’t be within the scope of this text, however if you need to be taught extra about it, right here is the GoodData.UI structure overview.
And only for completeness listed here are TypeScript dependencies:
npm set up --save-dev @varieties/react@^18.2.0 @varieties/react-dom@^18.2.0
Now that’s out of the way in which, let’s take a look at the code!
Step 2: Set setting variables
Be sure to have your .env and .env.native recordsdata with right values. After you clone the repository, you will notice a .env.native.template file within the /purchasers/sveltekit folder. It’s good to take away “template” from the filename in an effort to arrange every part accurately.
For .env, you’ll need to outline three variables:
# GoodData host
VITE_HOSTNAME=
# GoodData workspace id
VITE_WORKSPACE_ID=
# GoodData perception id
VITE_INSIGHT_ID=
If you happen to open a GoodData dashboard, you’ll find the HOSTNAME
and WORKSPACE_ID
within the URL:
https://<HOSTNAME>/dashboards/#/workspace/<WORKSPACE_ID>/dashboard/<DASHBOARD_ID>
For INSIGHT_ID
you’ll have to navigate to the Analyze tab after which navigate to one of many visualizations. There you’ll discover INSIGHT_ID
like this:
https://<HOSTNAME>/dashboards/#/workspace/<WORKSPACE_ID>/<INSIGHT_ID>/edit
For .env.native, you’ll need just one variable:
# GoodData API token
VITE_GD_API_TOKEN=
Examine Create an API token documentation for extra info.
In case you want to use this in your manufacturing, we extremely suggest to make use of OAuth, as you could possibly doubtlessly leak your API_TOKEN
.
Step 3: Arrange the Code
To rapidly embed GoodData Visualizations, you would wish to create a backend within the type of tigerFactory() in your chart part:
const backend = tigerFactory()
.onHostname(import.meta.env.VITE_HOSTNAME)
.withAuthentication(
new TigerTokenAuthProvider(import.meta.env.VITE_GD_API_TOKEN)
);
Then simply merely feed it with the onMounted()
methodology:
onMount(async () => {
const { InsightView } = await import('@gooddata/sdk-ui-ext');
const node = doc.getElementById('gooddata-chart');
const props = {
workspace: import.meta.env.VITE_WORKSPACE_ID,
perception: import.meta.env.VITE_INSIGHT_ID,
backend
};
if (node) {
ReactDOM.render(React.createElement(InsightView, props), node);
}
});
Now, you possibly can merely create <GoodDataChart>
and it’ll render the Visualization.
Here’s a quite simple template:
<h1>SvelteKit with GoodData</h1>
<GoodDataChart/>
Now you possibly can simply add styling and you’re set! Only for simplicity, let’s simply go together with a minimal one:
#gooddata-chart {
width: 100%;
peak: 400px;
}
Here’s a GitHub repo you possibly can simply begin with!
Step 4: Handle CORS
And that’s all for code! Fairly easy, is not it? 😉
Now the one factor that might be lacking is to care for CORS. That is fairly easy in GoodData:
- Navigate to your GoodData Occasion,
- Go to the settings
- Add allowed origins to the CORS.

Be aware: For detailed details about CORS, seek advice from the official documentation.
For my native growth, this was http://localhost:5173
:

Now simply merely run npm run dev
and it is best to see one thing like this:
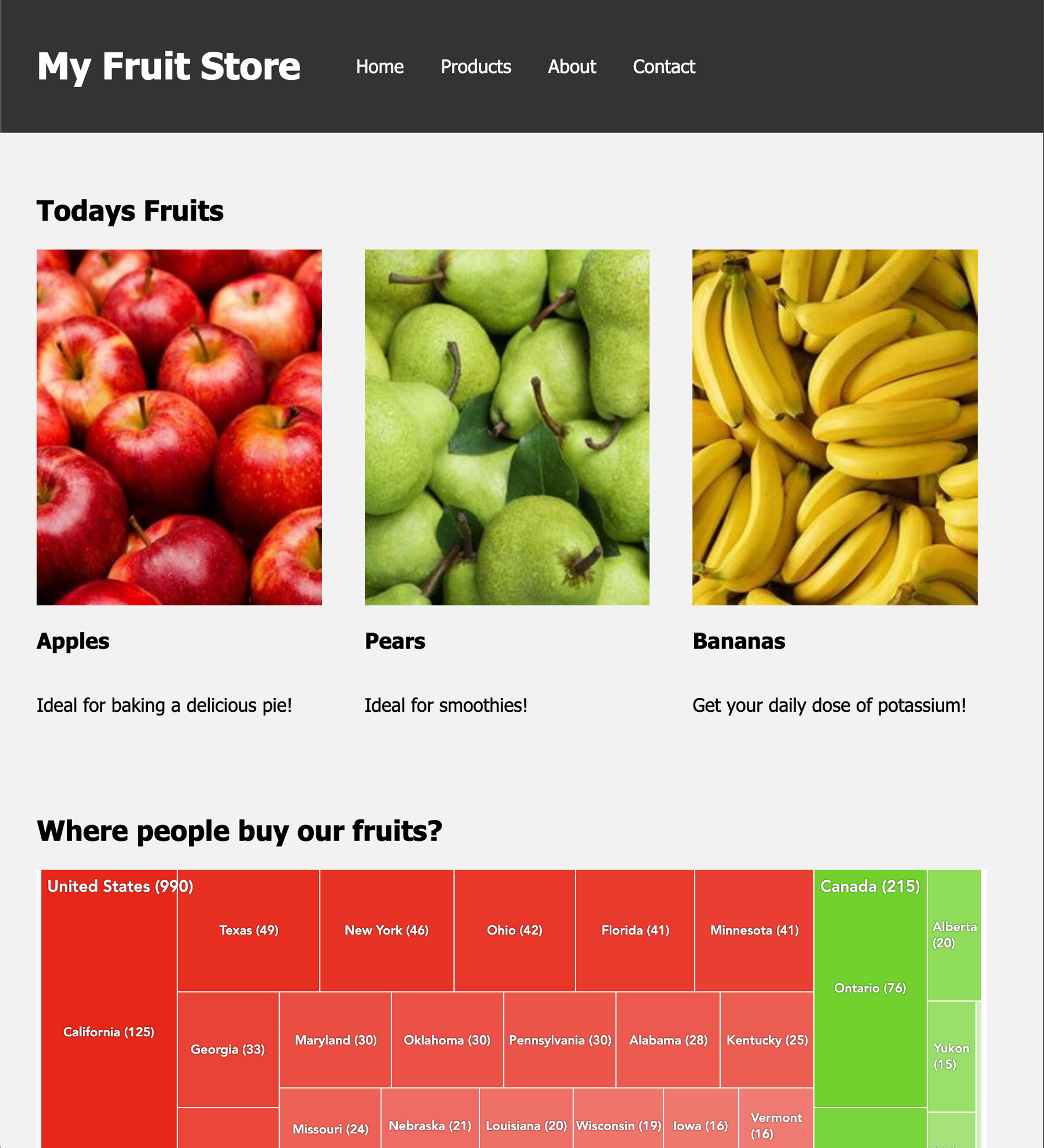
In case the colours of the visualization don’t fit your needs, you possibly can at all times change them.
Wish to Be taught extra?
If you wish to attempt GoodData, take a look at our free trial – it really works nicely with the GitHub repo! If you need to debate embedding, AI, dashboard plugins or no matter you’ve gotten in your thoughts, attain out to us on our group Slack.